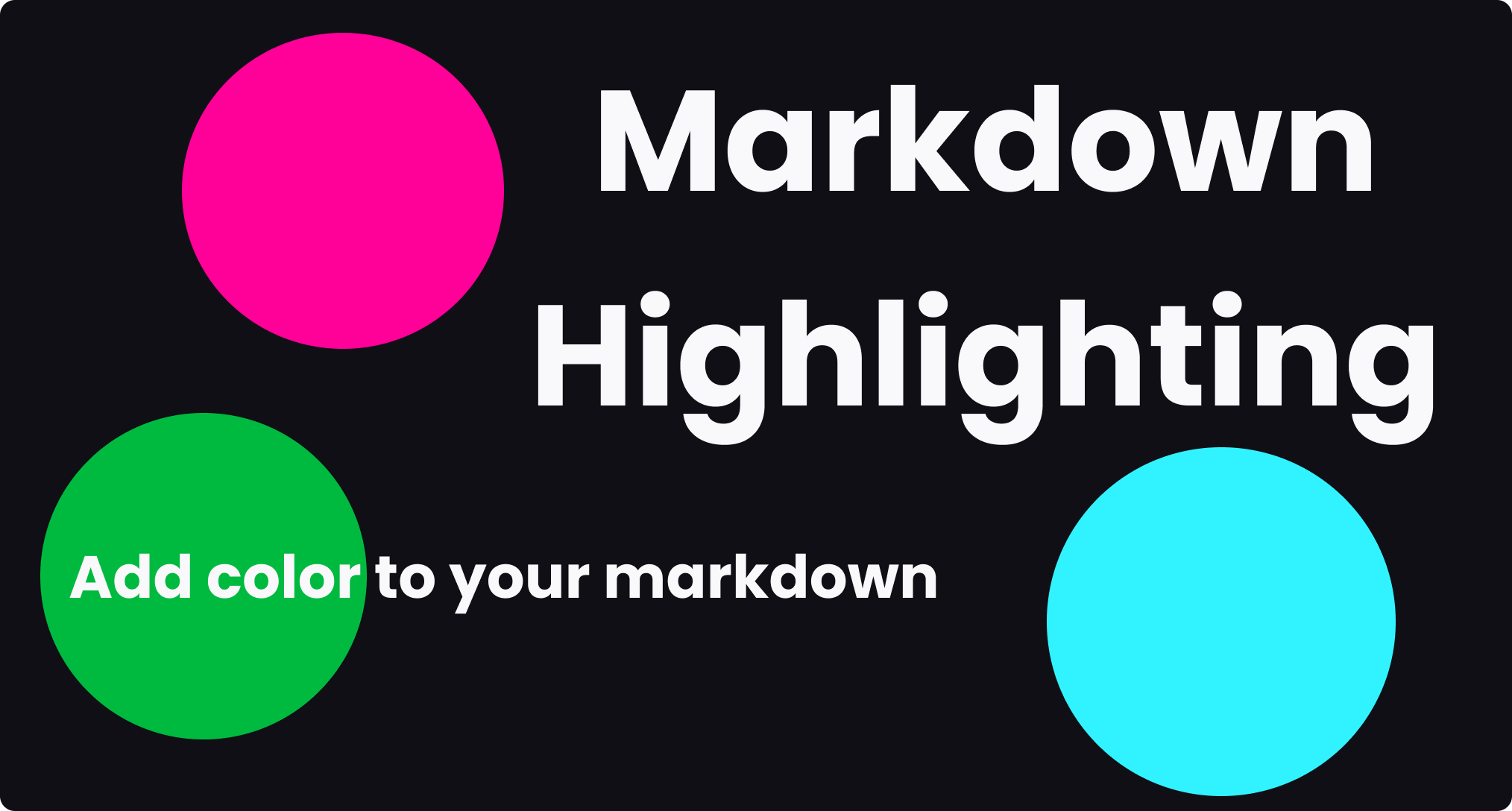
How to highlight markdown in ReactJS?
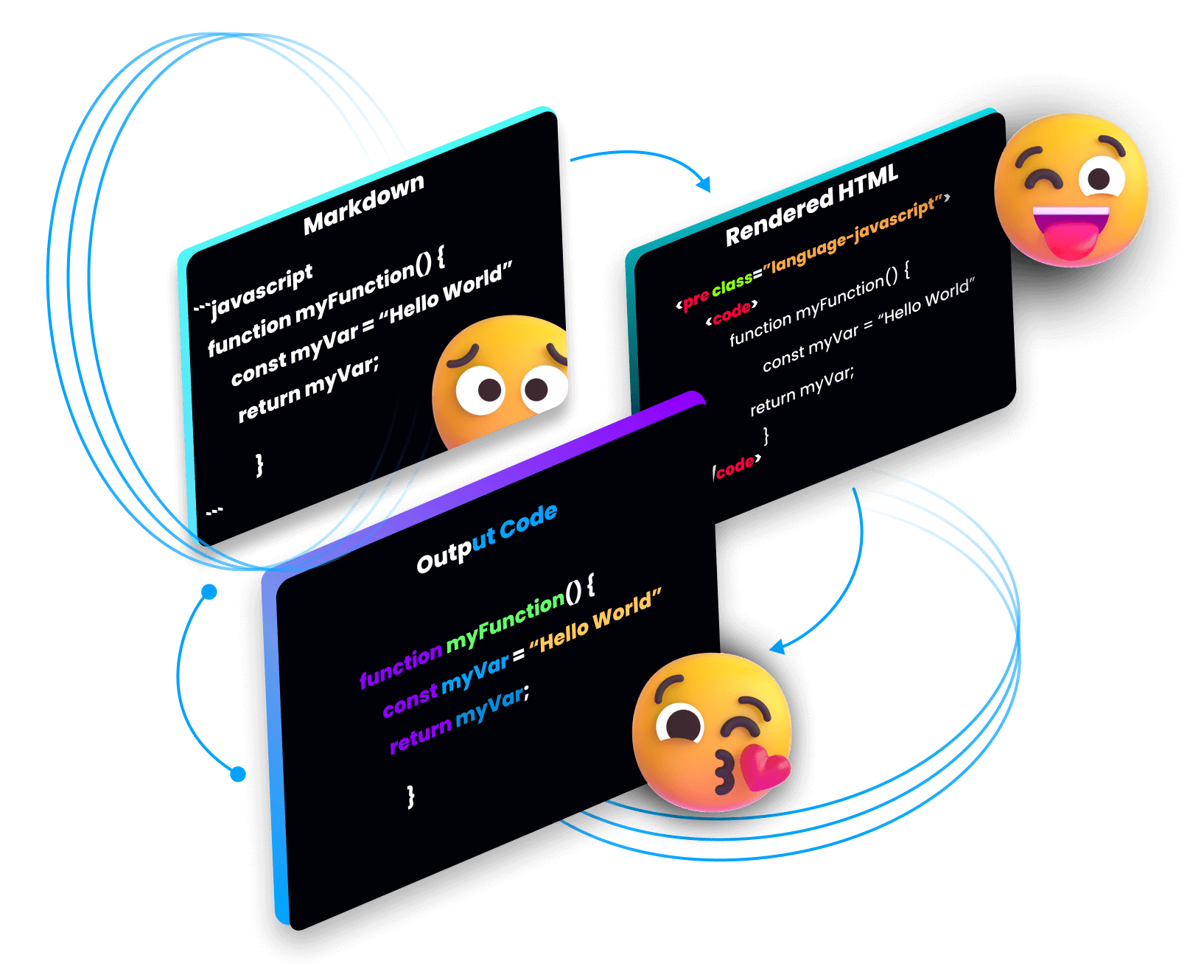
Highlighting your markdown can be very frastrating and annoying especially if your Code editor and a VS Extension like Markdown Previewer Enhanced hightlights your code perfectly when you use the standard ways.
There are a few ways to highlight Markdown the standard way is labelled in the Markdown Cheet Sheet, which is to state the language of your codeblock after your first three Backticks. Another way is to use a Highlighting library like Highlight.js or if you are using React JS you can use React-Highlight.js all these are an option and in this article I am going to show you how to use them.
Table of contents
- Styling Markdown with CSS
- Using markdown syntax
- Why is my browser rendering plaintext instead of highlighted code?
- How to use highlight.js in ReactJS
No one wants plain HTML on their website.
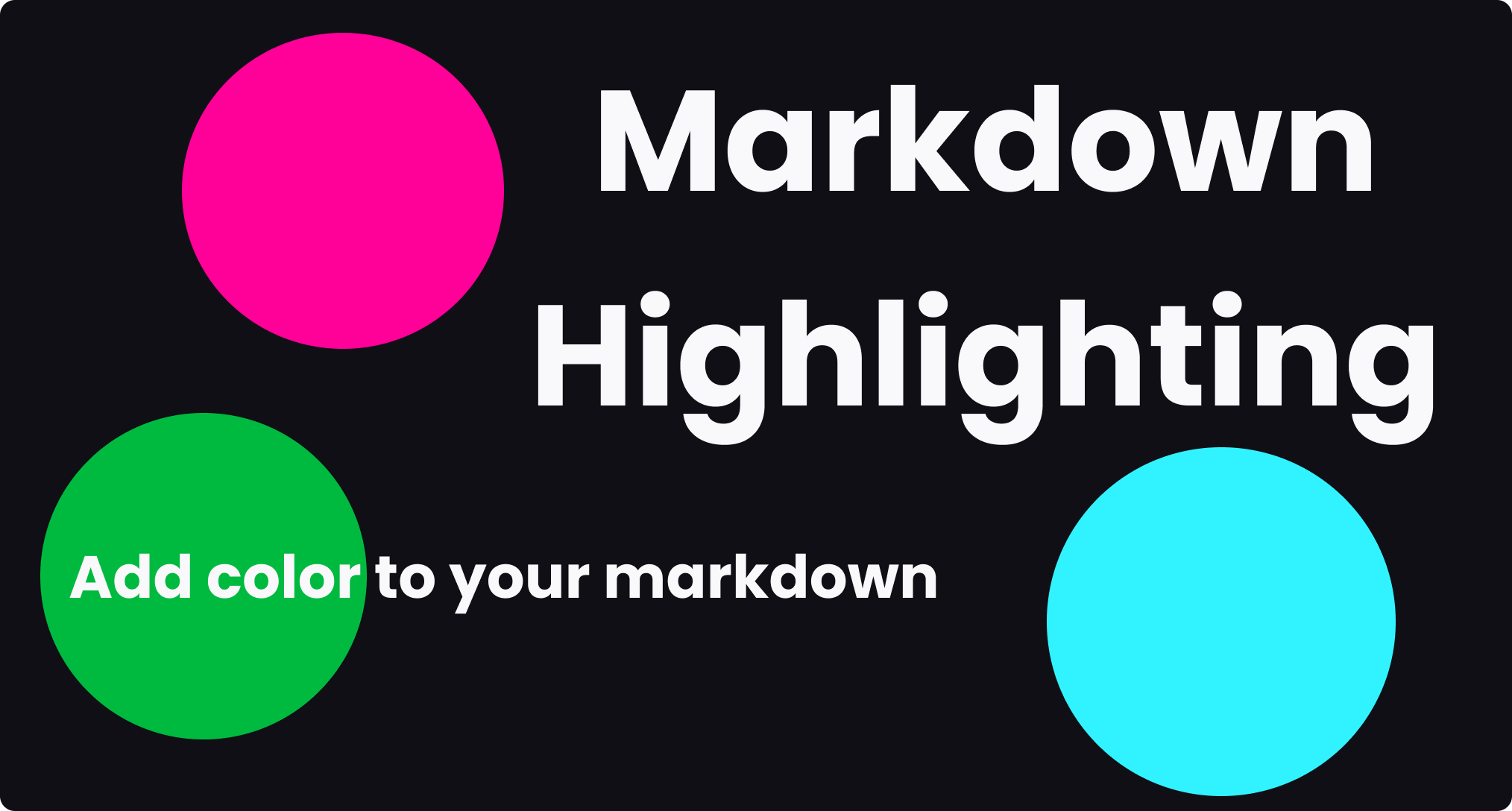
1. Styling markdown with CSS.
Markdown can be blend since it renders plain unstyled HTML, this is alright if you don't really care about styling and color, but if for any reason you want to style your markdown then it becomes a struggle if you don't really know where to even begin. There are ways to style the HTML rendered by markdown one is by refencing the HTML Tags markdown renders in your code.
code{
background: #0ff;
}
p{
color: red;
font-size: 0.6 em;
}
h1 {
font-family: "Arial", san-serif;
color: #fff;
}
This is fine and can work for most cases, but what happens when you want to style something like code that is where the problem starts. Although markdown has a pre-defined way to do this which is to state the language you want to use after the first three backticks (```) like how I did in the following block below.
2. Using Markdown Syntax
```javascript
function myFunction(){
alert("This code is highlighted in markdown");
}
```
This might work perfectly in your Markdown Previewer or Github, in most cases like in the browser this will not work and this is because the browser does not interpret Markdown but parses HTML, and this leads to misunderstandings, as the browser will not hightlight your code, and this is franstating.
In theory this is what should be rendered in your browser
function myFunction(){
alert("This code is highlighted in markdown");
}
But instead you are getting plaintext.
function myFunction(){
alert("This code is highlighted in markdown");
}
3. Why is my browser rendering plaintext instead of highlighted code?
Browsers were not made to render markdown but they render HTML, which means you need a markdown parser like marked.js to parse your markdown so it can be displayed in the browser.
Marked.js is a fast, lightweight and low level markdown compiler. It is able to run in both client-side and server-side projects as a command line interface (CLI).
To use marked.js you can install it from NPM or Yarn.
4. How to use highlight.js in ReactJS
$ npm install -g marked
#or
$ npm install marked
When you have your marked library installed you can then install your highlighting library. We are going to use highlight.js in this example, and to install it run.
$ npm install highlight.js
#or
$ yarn install highlight.js
After your hightlight.js has been installed now you can start highlighting your code.
import { marked } from 'marked'
import hljs from 'highlight.js';
import 'highlight.js/styles/dracula.css'; //This is the theme your code is going to be displayed with.
Now to put both libraries to use we can start writing some code.
import { marked } from 'marked'
import hljs from 'highlight.js';
import 'highlight.js/styles/dracula.css'; //This is the theme your code is going to be displayed with.
import { useEffect } from 'react'
export default function myFunction() {
let content = "# Your Markdown"
useEffect(() => {
function highlightCode() {
var pres = document.querySelectorAll("pre>code");
for (var i = 0; i < pres.length; i++) {
hljs.highlightElement(pres[i])
}
}
return highlightCode();
}, []);
return()
}
This is the easiest way to do this, the useEffect() will highlight your code when you first run the page and on every render. This makes sure your code is always highlighted without affecting perfomance.
There is a more advanced way to do this and it's based on the Marked library it is like this.
marked.setOptions({
renderer: new marked.Renderer(),
highlight: function(code, lang) {
const hljs = require('highlight.js');
const language = hljs.getLanguage(lang) ? lang : 'plaintext';
return hljs.highlight(code, { language }).value;
},
langPrefix: 'hljs language-', // highlight.js css expects a top-level 'hljs' class.
pedantic: false,
gfm: true,
breaks: false,
sanitize: false,
smartLists: true,
smartypants: false,
xhtml: false
});
export default function myFunction() {
let content = "# Your Markdown"
return()
}
You can find this code on the Marked Library Documentation.
This is the JSX you should return in your component.
<>
{/*Remember to sanitize your markdown to prevent attacks.*/}
<div dangerouslySetInnerHTML={{__html: DOMPurify.sanitize(marked.parse(content))}}></div>
</>
5. Conclusion
There are many ways to higlight markdown in your browser both with React or just with HTML where you can use Scripts. Really the possibilities are endless you just need to read documentation of the different frameworks and you will be fine, or just follow this blog for an easier approach if you feel a bit lazy like most of us sometimes.