function A(ps){
c = []
sd = 0.05
ld = .1
ps.forEach(e => {
dtot = 0
if(e > 5000){
dtot = e * d
c.push(dtot)
}
})
}
Why is code splitting an important skill to learn for every developer?
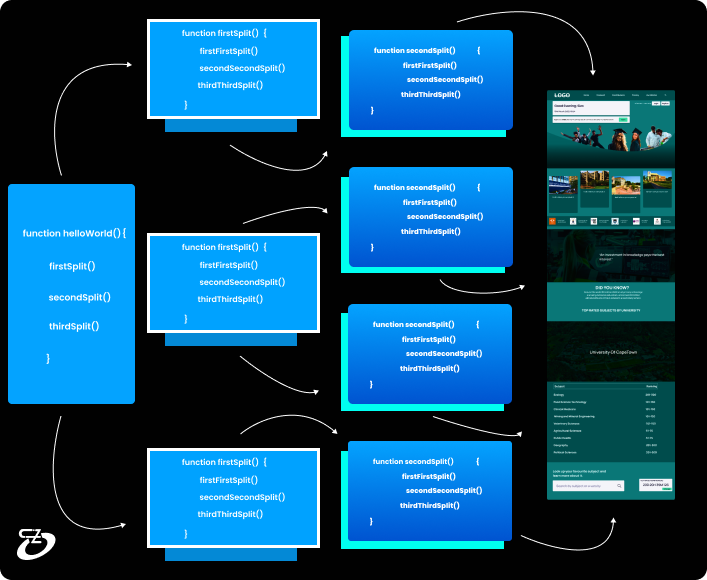
Overview
Coding is fantastic, and writing code is the most intriguing thing one can do while also solving problems. However, writing code involves more than just creating line after line of code and making your projects work as you intended.
There is more between the lines, and that is clear and readable code. As someone once said, "Do not comment on bad code; rewrite it." This may sound obvious, but most developers overlook the fundamentals of coding. In this article, I will define bad code and explain how code splitting can help you improve the code you write.
1. What is bad code?
Bad code is described as hastily written, unmaintainable code. I refer to it as "Me Code", meaning only I, the developer or writer, can read or understand what each line does. This is particularly terrible since, in addition to the fact that no one else can read it, you might also forget what you were trying to accomplish if you come back to it later.
When writing code, it's important to be thorough and take maintainability into account. Will you be able to update the code? Or will it be practically impossible due to how difficult it will be? Making sure that your code is documented for both you and others is also essential.
But the fact still remains that documenting bad code is just like riding a horse without a saddle; it's bad for your ass. So cover your butt by taking the time to design the code first before writing it. Establish what you want to accomplish, and then write the code.
2. What makes good code?
Readability and maintainability. There's nothing more to it; it's just about asking yourself, Will someone else be able to maintain the code I'm writing? Will I be able to read it in the future? After you have answered those questions, start writing comments on your code, documenting what each line does.
The way you know that code is well written and documented is by giving it to a beginner and letting them try to tell you what it does. If they are able to somewhat understand what it's supposed to do, then you've written great code.
Good code is like poetry; each line flows seamlessly into the next, and even a non-programmer can read it, just as people who are not poets can read and understand poetry.
A basic example of bad code;
Good code;
// Calculate discounts based on prices
function calculateDiscounts(pricesList){
updatedPricesList = []
smallestDiscount = 0.05
largestDiscount = 0.1
pricesList.forEach(price => {
calculatedDiscount = 0
if(price > 5000){
calculatedDiscount = price * smallestDiscount
updatedPricesList.push(calculatedDiscount)
}
})
return updatedPricesList
}
The logic doesn't have to be the greatest, but if the code describes itself, then that is good code, other than someone having to deal with code that is filled with a bunch of single-letter variables that make no sense.
What is code splitting?
Code splitting is the process of breaking up your code into digestible bits. Although there is more to code splitting, I only want to cover the bare minimum in this article. This is to help developers, both new and experienced, who may not be familiar with the notion.
By creating distinct files in your folder tree that are then available via exporting and importing to and from other files, code splitting allows you to reduce the size of your code bundle. The majority of languages, including JavaScript, Python, C++, and others, support this.
Multiple files containing various portions of your code that, when merged, finish your project would be used instead of one very large file containing all of your code.
To use import in JavaScript;
import FunctionName from "./fileWithFunction"
This is especially used with FrontEnd frameworks like ReactJS, Gatsby and others.
Another method used in NodeJS Module Files
const FunctionName = require("./fileWithFunction")
Many languages allow you to import external files into other files, and it is critical that you be able to separate your code. This is useful when you want to update a specific section of your project without disrupting the overall flow of the project. Aside from not disrupting the flow of your project, you can also read and maintain your code, readily spotting issues that you might otherwise overlook.
Exporting is also similar (JavaScript);
function FunctionName(){
return
}
export default FunctionName;
Another way;
function FunctionName(){
return
}
module.exports = FunctionName;
Now that you are able to import and export functions, you are able to split your code. You can even go as far as to put each function in its own file or put related functions in a separate file and use them on demand.
Why is code splitting often overlooked?
This happens mostly to beginners or people who have never built a large app. Before you build something big, it's really not necessary to split your code, but as your code grows, you start to notice how important it is.
As your code base grows, it becomes harder and harder to maintain and find bugs. The larger your code file, the bigger the headache you're going to get.
Conclusion
Code splitting is dividing your code into chunks, putting it into different files, and importing and exporting them as needed. It is very important to start practicing splitting your code, even in a small project, just so you can get a basic understanding of what code splitting is and how it works.
Also, practice writing good and readable code and documenting it as much as you can.