import smtplib
# Create the SMTP object
smtpObj = smtplib.SMTP_SSL('smtp.gmail.com', 465)
Send emails with Python using SMTP Server
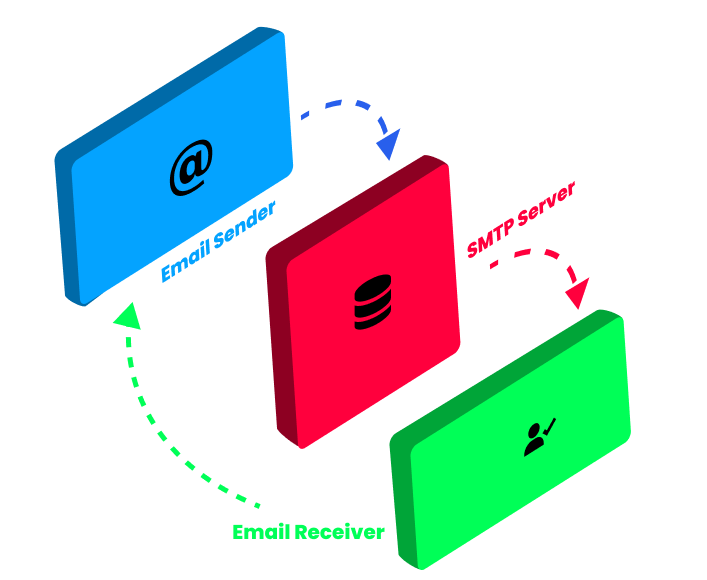
Although services like Outlook, MS Word and Others that make the sending of bulk emails easier for their customers, have simplified the setting up of email reminders, It still bugs developers when it comes to intergrating the same type of systems in their apps. What if I told you there is an easier and not so costly way to send emails to your users directly from your source code with Python.
In this article I am going to cover how to send emails with Python and give code examples that will help a lot of programmers that don't know where to begin sending emails, especially if it's just a portfolio project that you want to send emails from.
1. What is SMTP?
Simple Mail Transfer Protocol (SMTP) is a protocol used to send emails across the internet. SMTP specifies how emails should be structured, formatted, encrypted and sent between mail servers. There are a lot of details that happen behind the scenes of this process, but in this article we will cover the simplified version, and Python's module smtplib simplifies this process so that it is convenient for every developer to use the protocol.
2. How to connect to an SMTP server?
Email providers have different ways to allow you to connect to the SMTP server and they all have different ports to connect to. Usually for different providers there are different domain names for the SMTP server, eg. smtp.providername.com, as you can see the domain is prefixed with the smtp keyword.
Port 587 is mostly used as it is the port used by the common encryption standard, TLS.
After you've searched and found a domain name e.g Gmail's, smtp.gmail.com and it's respective port number. Now create an object by calling stmplib.SMTP(),
Note: If the stmplib.SMTP() call does not work try smtplib.SSL_SMTP(), this may mean your server does not support TLS.
Lets start by connecting to the server
This will connect you to the Gmail SMTP server and then we can move on to the second step.
Before any other method though you need to call the ehlo() method on the object you created to establish a connection to the server.
Send Hello to the server
This step is necessary for the connection to the server and you can do this by calling the ehlo() method on the object we created. The greeting is successful if you get back a 250 code from the server. Another important note is to make sure you call ehlo() right after creating your object, because if you don't the other methods will throw errors.
import smtplib
# Create the SMTP object
# smtpObj = smtplib.SMTP_SSL('smtp.gmail.com', 465)
smtpObj = smtplib.SMTP('smtp.example.com', 587) # Notice we change the domain and port number
# Call the ehlo() method after the object first
smtp.ehlo()
# (220, b'2.0.0 Ready to start TLS')
What is TLS encryption
Transporting Layer Security (TLS) is a cryptographic protocol designed to provide communications security over a computer network. The protocol is widely used in applications such as email, instant messaging, and voice over IP, but its use in securing HTTPS remains the most publicly visible.
The TLS is associated with the port 587, if you are using this port that means you are using the TLS encryption, but it is not automatically enabled like the SSL encryption. To enable is in your project you'll need to run the starttls() function.
Enabling the TLS encryption.
Note: In our code above we used SSL encryption so we don't need to call the starttls() method, but for clarity we will use it in this example.
import smtplib
# Create the SMTP object
# smtpObj = smtplib.SMTP_SSL('smtp.gmail.com', 465)
smtpObj = smtplib.SMTP('smtp.example.com', 587) # Notice we changed the domain and port number
# Call the ehlo() method after the object first
smtp.ehlo()
smtpObj.starttls()
# (220, b'2.0.0 Ready to start TLS')
Now that you are connected to the server and protected you can login with your provider credentials, keep these private.
Log in to the server
For Gmail your username will be your Email Address and your password will be your Email Password Pass a string of your email address as the first argument and a string of your password as the second argument. The 235 in the return value means authentication was successful. Python will raise an smtplib .SMTPAuthenticationError exception for incorrect passwords
import smtplib
# Create the SMTP object
# smtpObj = smtplib.SMTP_SSL('smtp.gmail.com', 465)
smtpObj = smtplib.SMTP('smtp.example.com', 587) # Notice we change the domain and port number
# Call the ehlo() method after the object first
smtp.ehlo()
smtpObj.starttls()
# Use you email and email password to log in...
smtpObj.login('my_email_address@gmail.com', 'MY_SECRET_PASSWORD')
4. Sending an Email
Now that we are done with the necessities we can do what we came here for. Sending an email. To send an email you will need to call the sendmail() method which takes in 3 arguments.
- Your Email Address.
- The Recipient's email.
- The Email Subject and Message in one string.
The body of the email should look something like this, "Subject: Sending an email \n", the \n at the end is to add a new line after the subject of the email.
If for some reason the sending fails, sendmail() will return a dictionary containing the failed emails. If the emails have been succesfully sent the dictionary returned will be empty, {}.
import smtplib
# Create the SMTP object
# smtpObj = smtplib.SMTP_SSL('smtp.gmail.com', 465)
smtpObj = smtplib.SMTP('smtp.example.com', 587) # Notice we change the domain and port number
# Call the ehlo() method after the object first
smtp.ehlo()
smtpObj.starttls()
# Use you email and email password to log in...
smtpObj.login('my_email_address@gmail.com', 'MY_SECRET_PASSWORD')
# Send email
smtpObj.sendmail('my_email_address@gmail.com', 'recipient@example.com',
'Subject: Sending Email\n Dear Sizo Develops Team,
I have read your article and this is where I got.
Sincerely, Reader\'s Name')
Now we are done with the sending of emails and setting up of the SMTP server, all that's left to do is disconnect from the server. To disconnect from the server you need to call the quit() method. and then you are done.
The entire code without comments will look like this.
import smtplib
smtpObj = smtplib.SMTP('smtp.example.com', 587)
smtp.ehlo()
smtpObj.starttls()
smtpObj.login('my_email_address@gmail.com', 'MY_SECRET_PASSWORD')
smtpObj.sendmail('my_email_address@gmail.com', 'recipient@example.com',
'Subject: Sending Email\n Dear Sizo Develops Team,
I have read your article and this is where I got. Sincerely,
Reader\'s Name')
smtpObj.quit()
Now go ahead and try it yourself.
5. Conclusion.
Python is a really powerful language, although you can do this in any language you want, Python is by far the most straight forward language when it comes to automation of repeating tasks, in my opinion. Feel free to try different ways to do this task, as that is the beauty of programming, there are many solutions to one problem.