{
name: "Sizo Develops"
age: 24
country: "South Africa"
}
Restful APIs: A beginner's guide to REST APIs.
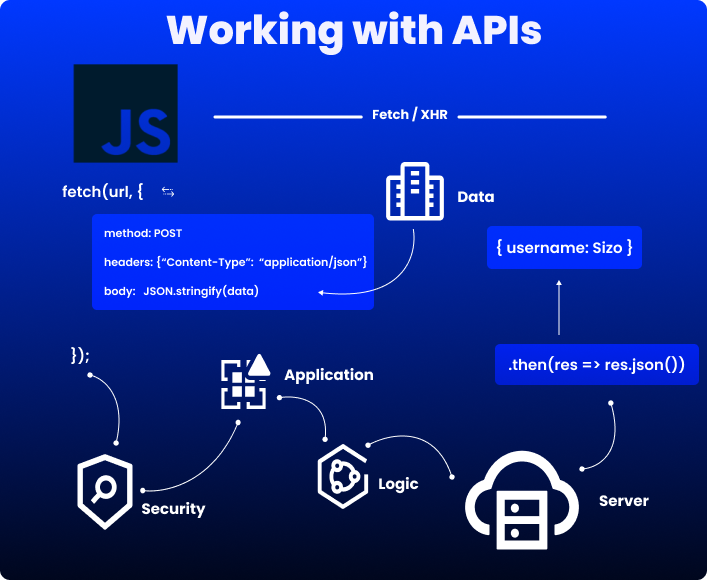
Overview
A REST API is an API that follows and conforms to the design principles of the REST Architectural style, it was first defined in the year 2000 by Dr. Roy Fielding in his doctoral dissertation, and has now emerged to be one of the primarily used API methods to connect components and applications in a microservice architecture, this is due to its high level of flexibility and freedom for developers.
What is an API?
The application programming interface (API) is a set of rules that define how applications or devices can connect and or communicate with one another. It is a method of securely exchanging information between two computer systems over the internet. An API connects you, the user (the app you are using), to the information you seek online. Businesses use internal APIs to automate invoicing and generate payslips, among other things.
What is REST?
Representational State Transfer (REST) is a software architecture that controls and sets conditions of how an API should work, A web service that makes use of the REST API is generally regarded as RESTful. This type of software architecture was created as a guideline to manage communications over the internet.
The RESTful API has multiple principles that comprise its architectural style, and these include but are not limited to:
Statelessness
Statelessness is a communication method in the REST architecture in which the server completes each client request independently of previous requests, implying that no data is stored between client requests.
Cacheability
Caching is the process of storing some responses on the client or server in order to improve server response time and increase server scalability. RESTful API responses must include information on whether or not caching is permitted for the delivered resource.
Uniform Interface
A fundamental standard for the design of RESTful web services is the uniform interface. It implies that the server sends data in a standard format; the formatted resource is referred to as a representation in REST. Regardless of where the request is coming from, all API requests for the same resource should look the same.
Layered system
Client and server responses in RESTful APIs pass through multiple layers; you should never assume that the server and client applications connect directly to each other. An API can be designed to use multiple layers, such as security, application, and business logic, to fulfill client requests. Neither the client nor the server can tell whether they are communicating with the end application or an intermediary.
How do REST APIs work?
RESTful APIs communicate via HTTP requests to perform standard database operations, like Creating, Reading, Updating, and Deleting records from the database, (also known as CRUD). The HTTP methods that are commonly used with REST APIs are,
GET - in order to retrieve a record or entries from a server.
POST - this is for adding new records.
PUT - this is used to update existing records.
DELETE - as the name says, it deletes records from the database
HTTP headers - this typically contains information on the request, such as the request status or the format of the response and request.
RESTful API resources are often returned as XML or JSON, and the developer can transform this data into a JavaScript Object Notation (JSON) format. JSON is the most often used format for APIs because it makes data easy to organize and comprehend.
Although JSON is the most often utilized, alternative forms like Python, HTML, XLT, PHP, and Plain Text are also available.
The response of an API call contains the body, a status line and headers.
Message Body
The body of a response typically looks like this;
Take note of the curly brackets, which wrap around objects in JS to denote JSON. These can contain any JavaScript data type, such as numbers, arrays, strings, and even objects.
Status Line
The status line comprises a three-digit code from the HTTP status codes; these codes reflect the type of response the API produces, which can be either;
2XX Which indicates that the request was successful.
4XX and 5XX Which indicates a failure.
3XX These indicate a URL redirect.
The examples are;
200 success
201 POST method successful
400 incorrect request
404 resource not found
Headers
As previously said, the headers provide information about the response.
A Headers object has an associated header list, which is initially empty and consists of zero or more name and value pairs.
// To create a Headers object
const headers = new Headers()
Headers have multiple methods that help manipulate the data that goes into them.
Header Methods
headers.append(name, value) - Appends a header to headers.
headers.delete(name) - Removes a header from headers.
headers.get(name) - Returns as a string the values of all headers whose name is name, separated by a comma and a space.
headers.has(name) - Returns whether there is a header whose name is name.
headers.set(name, value) - Replaces the value of the first header whose name is name with value and removes any remaining headers whose name is name.
There are two methods for making an API call in JavaScript: the Fetch API and XMLHttpRequest, which are the most widely utilized.
Fetch API
The Fetch API provides a resource retrieval interface. Unlike an XMLHttpRequest, Fetch will not reject on an HTTP error, which might result in a 404 or 500 response, but will instead return properly with (ok set to false). Fetch only rejects requests if the network fails or if anything prevents the request from being fulfilled.
Fetch accepts one mandatory parameter, the URL or path of the resource to be fetched, and returns a Promise that resolves into a response, indicating that it is an asynchronous function. You may also supply an init object as the second parameter, which holds additional information about the request, such as the Headers.
A basic fetch request is really easy to set up.
fetch(url)
.then(res => res.json())
.then(data => {
console.log(data)
})
This code retrieves a resource from the url's supplied path and writes it to the console. This is the most basic type of fetch, taking simply the path to the resource and returning a promise. Keep in mind that the promise response is not JSON, but rather a representation of the entire HTTP response; to obtain the JSON, we must use the json() function.
The init Object
Optionally, you can pass in the init object as the second argument to fetch, which controls a few other things.
// Adding a new entry to my profile path.
data = {
name: "Sizo Develops"
age: 24
country: "South Africa"
}
fetch("http://example.com/profile", {
method: POST,
mode: "cors",
cache: "no-cache",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(data)
})
.then(res => res.json())
.then(data => {
console.log(data)
})
As you can see, we are using the HTTP method POST to create a user in the profile path; if we now use API on the profile path, we will find the newly generated user. The benefit of APIs is that you can add data programmically if they allow it.
XMLHttpRequest
To communicate with servers, XMLHttpRequest (XHR) objects are utilized. Despite the fact that it says XML, you can still obtain any form of data you need, just like fetch, and all recent browsers have XMLHttpRequest built in, making it easily accessible to you.
With XHR, you may update a web page without refreshing it, transmit data in the background, request data, or send data to a server after the page has loaded.
The XHR object has multiple methods;
XMLHttpRequest.abort() - Aborts the request.
XMLHttpRequest.getResponseHeader() - Returns a string containing the text of the provided header, or null if the answer has yet to be received or the header does not exist in the response.
XMLHttpRequest.setRequestHeader() - Sets the value of an HTTP request header. You must call setRequestHeader() after open(), but before send().
XMLHttpRequest.open() - Initializes the request.
XMLHttpRequest.send() - Sends the request.
The basic syntax for XHR is;
const request = new XMLHttpRequest()
request.open("GET", url)
request.send()
As you can see, we initiated and submitted the request but did not process the response. To handle XHR responses, you may use event listeners or the XMLHttpRequest.onreadystatechange property.
Using an event listener;
textElement = document.getElementById("text")
function setTextElement(){
textElement.innerHTML = this.responseText
}
const request = new XMLHttpRequest()
request.addEventListener("load", setTextElement)
request.open("GET", url)
request.send()
Remember how we stated XHR may refresh a page and make requests to the server after it has loaded? This is what the code above does. Another XHR property that returns the raw HTML from the response is XMLHttpRequest.responseText; this is why we used innerHTML to inject the raw HTML into our page.
Using the XMLHttpRequest.onreadystatechange property.
textElement = document.getElementById("text")
function setTextElement(){
textElement.innerHTML = this.responseText
}
const request = new XMLHttpRequest()
request.addEventListener("load", setTextElement)
request.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
setTextElement()
}
};
request.open("GET", url)
request.send()
The above code creates an event that checks to see whether a state has changed; the XMLHttpRequest.onreadystatechange event is triggered when the state of the request provided changes. If this.readyState is 4 and this.status is 200, that signifies the response is ready and you may use it.
XMLHttpRequest extends two other popular utilities, Axios and Ajax, although we will not cover them in this post.
As you may have noticed, both of our previous code examples assumed that the request we sent would return a success status, but what if the request fails? That's where error handling comes into play. As an API user, you have no way of knowing what problems may develop while your request is being processed; to prevent this, Fetch has the Catch() function, which catches any exceptions and throws an error.
To white a catch function with fetch.
fetch(url)
.then(res => res.json())
.then(data => data)
.catch(error => {
console.log(error)
});
Even if you know the outcome of the request ahead of time, the catch() function is quite important to add since it allows you to do a variety of things such as not just report the error to the console but also use a fallback if the response fails.
You may use this to do some cool things, such as layout changes or animations, depending on what you're working on.
.catch(error => {
someElement.innerText = "Oops Looks like you've messed up!"
});
The error property or an event listener are used to handle errors in XHR.
request.onerror(error => { DO MAGIC })
// OR
request.addEventListener("error", someFunction)
Within the onreadystatuschange function, you can also use an else statement.
There are other ways to handle errors in JavaScript that can be combined with these two methods depending on the needs of your project.
Consider the Try-Catch approach.
We've now covered a lot about APIs, including how they function and how to work with them.
What can you do with API?
APIs are the backbone of practically all applications; to conserve space and resources, you may want store your data somewhere in a database and use an API to access and make us of that data. There are several APIs available, some of which are free and others of which cost subscriptions; nonetheless, anything can now be converted into an API to aid in the development of useful APPS.
Free APIs you can use to build your Apps
Spotify API
Youtube API
TMDb API
Deezer API
Weather API
Covid Cases API
There are many free apis available; these are just a handful that are simpler to use when developing web apps.
Happy Coding!!!